How Developers Can Enhance Their Debugging Skills By Gustavo Woltmann
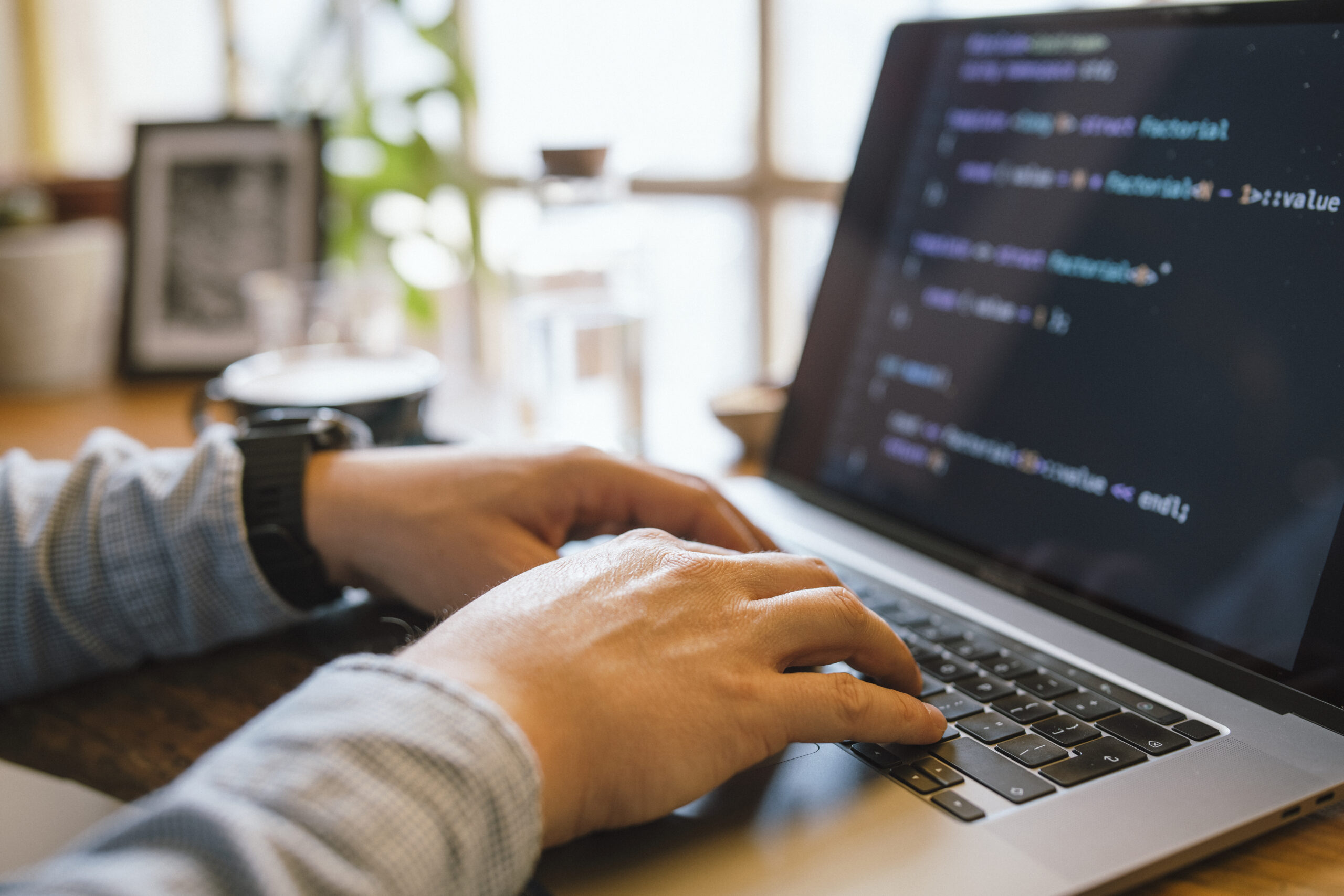
Debugging is Just about the most critical — however frequently neglected — competencies inside a developer’s toolkit. It is not almost correcting broken code; it’s about knowledge how and why matters go wrong, and Understanding to Consider methodically to unravel problems proficiently. Irrespective of whether you are a beginner or even a seasoned developer, sharpening your debugging expertise can help you save several hours of frustration and dramatically enhance your productivity. Listed here are numerous techniques that will help builders stage up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of several quickest methods builders can elevate their debugging competencies is by mastering the applications they use everyday. When producing code is one particular Portion of improvement, understanding how to connect with it proficiently through execution is equally significant. Present day advancement environments come Geared up with potent debugging abilities — but quite a few developers only scratch the area of what these instruments can do.
Take, such as, an Built-in Improvement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources permit you to established breakpoints, inspect the worth of variables at runtime, phase by way of code line by line, and in some cases modify code about the fly. When applied appropriately, they Allow you to observe specifically how your code behaves throughout execution, which happens to be a must have for monitoring down elusive bugs.
Browser developer tools, including Chrome DevTools, are indispensable for entrance-stop developers. They assist you to inspect the DOM, keep an eye on network requests, look at genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can change disheartening UI concerns into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage in excess of running processes and memory management. Discovering these tools could have a steeper Mastering curve but pays off when debugging overall performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become cozy with Model Regulate units like Git to know code historical past, discover the exact second bugs ended up released, and isolate problematic variations.
Ultimately, mastering your resources implies likely further than default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting making sure that when difficulties crop up, you’re not shed at the hours of darkness. The greater you are aware of your tools, the greater time you can spend resolving the particular challenge in lieu of fumbling by the procedure.
Reproduce the situation
Among the most essential — and sometimes disregarded — actions in effective debugging is reproducing the problem. Right before leaping to the code or generating guesses, developers need to produce a reliable setting or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of prospect, typically leading to squandered time and fragile code alterations.
The first step in reproducing a problem is collecting as much context as feasible. Check with queries like: What steps brought about The difficulty? Which natural environment was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you have got, the less complicated it gets to be to isolate the precise situations less than which the bug happens.
Once you’ve gathered enough facts, make an effort to recreate the condition in your local ecosystem. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, look at writing automatic exams that replicate the sting cases or condition transitions included. These assessments not only aid expose the condition but additionally protect against regressions in the future.
Often, The difficulty might be setting-unique — it might take place only on sure operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical technique. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. Having a reproducible situation, You need to use your debugging instruments additional proficiently, exam opportunity fixes properly, and connect more Evidently using your crew or end users. It turns an summary grievance into a concrete challenge — and that’s where builders thrive.
Read and Understand the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. In lieu of observing them as annoying interruptions, developers should learn to take care of mistake messages as direct communications from the system. They normally inform you what exactly occurred, where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the concept cautiously As well as in entire. Quite a few developers, specially when underneath time stress, look at the primary line and right away start building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like yahoo — read and fully grasp them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line variety? What module or function activated it? These questions can information your investigation and level you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Mastering to acknowledge these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s essential to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger challenges and provide hints about probable bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood without having to pause execution or action from the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging stages involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information during enhancement, Facts for normal functions (like profitable commence-ups), WARN for opportunity challenges that don’t split the application, ERROR for genuine troubles, and FATAL when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure vital messages and decelerate your method. Focus on critical functions, state variations, input/output values, and critical decision details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments the place stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a nicely-imagined-out logging solution, you'll be able to lessen the time it requires to identify problems, get deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a complex undertaking—it is a type of investigation. To properly identify and resolve bugs, builders ought to approach the process like a detective fixing a thriller. This mentality helps break down sophisticated troubles into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting proof. Consider the signs or symptoms of the trouble: error messages, incorrect output, or functionality difficulties. Identical to a detective surveys against the law scene, obtain just as much relevant information as you are able to without having leaping to conclusions. Use logs, exam conditions, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Question by yourself: What may be triggering this habits? Have any adjustments not too long ago been created towards the codebase? Has this problem happened right before less than very similar situation? The purpose is usually to slim down prospects and determine potential culprits.
Then, test your theories systematically. Make an effort to recreate the trouble in a managed setting. Should you suspect a specific purpose or element, isolate it and verify if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you closer to the reality.
Spend shut focus to small facts. Bugs usually disguise while in the least predicted places—just like a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface afterwards.
Finally, continue to keep notes on Everything you tried out and discovered. Equally as detectives log their investigations, documenting your debugging system can preserve time for upcoming problems and support others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more effective at uncovering hidden concerns in advanced systems.
Compose Assessments
Crafting tests is one of the most effective strategies to help your debugging skills and All round growth performance. Tests not just support capture bugs early and also function a security Web that offers you confidence when creating improvements towards your codebase. A well-tested application is easier to debug because it enables you to pinpoint precisely in which and when a difficulty happens.
Begin with unit exams, which concentrate on personal functions or modules. These little, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know the place to seem, substantially lowering the time spent debugging. Device assessments are Specially beneficial for catching regression bugs—concerns that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclusion-to-conclude exams into your workflow. These help make sure various aspects of your software function alongside one another efficiently. They’re specifically helpful for catching bugs that manifest in intricate methods with multiple parts or providers interacting. If something breaks, your assessments can tell you which Element of the pipeline failed and less than what problems.
Creating checks also forces you to Assume critically about your code. To check a function thoroughly, you may need to know its inputs, predicted outputs, and edge instances. This standard of knowing The natural way qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the exam fails constantly, you may concentrate on repairing the bug and check out your check move when The difficulty is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable system—assisting you catch far more bugs, a lot quicker and a lot more reliably.
Choose Breaks
When debugging a tough problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are far too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just several hours previously. In this particular condition, your brain gets to be considerably less productive at difficulty-solving. A short wander, a espresso split, and even switching to a special job for 10–quarter-hour can refresh your emphasis. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious do the job from the qualifications.
Breaks also assist prevent burnout, Primarily through more time debugging sessions. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality and a clearer mentality. You could possibly abruptly notice a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a very good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that time to move around, stretch, or do a little something unrelated to code. It could experience counterintuitive, Specially under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Each individual bug you encounter is much more than simply A short lived setback—it's a chance to increase to be a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you a thing important in the event you take some time to mirror and assess what went Completely wrong.
Start by asking on your own a few important concerns after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught before with improved tactics like device tests, code assessments, or logging? The solutions typically expose blind places with your workflow or knowledge and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing Anything you've uncovered from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or A fast more info information-sharing session, helping Many others stay away from the exact same issue boosts staff efficiency and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but individuals that constantly master from their blunders.
Eventually, Each and every bug you take care of adds a different layer for your ability set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — but the payoff is big. It would make you a far more effective, self-confident, and able developer. Another time you're knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.